Get Timestamp from Date - The Ultimate Guide
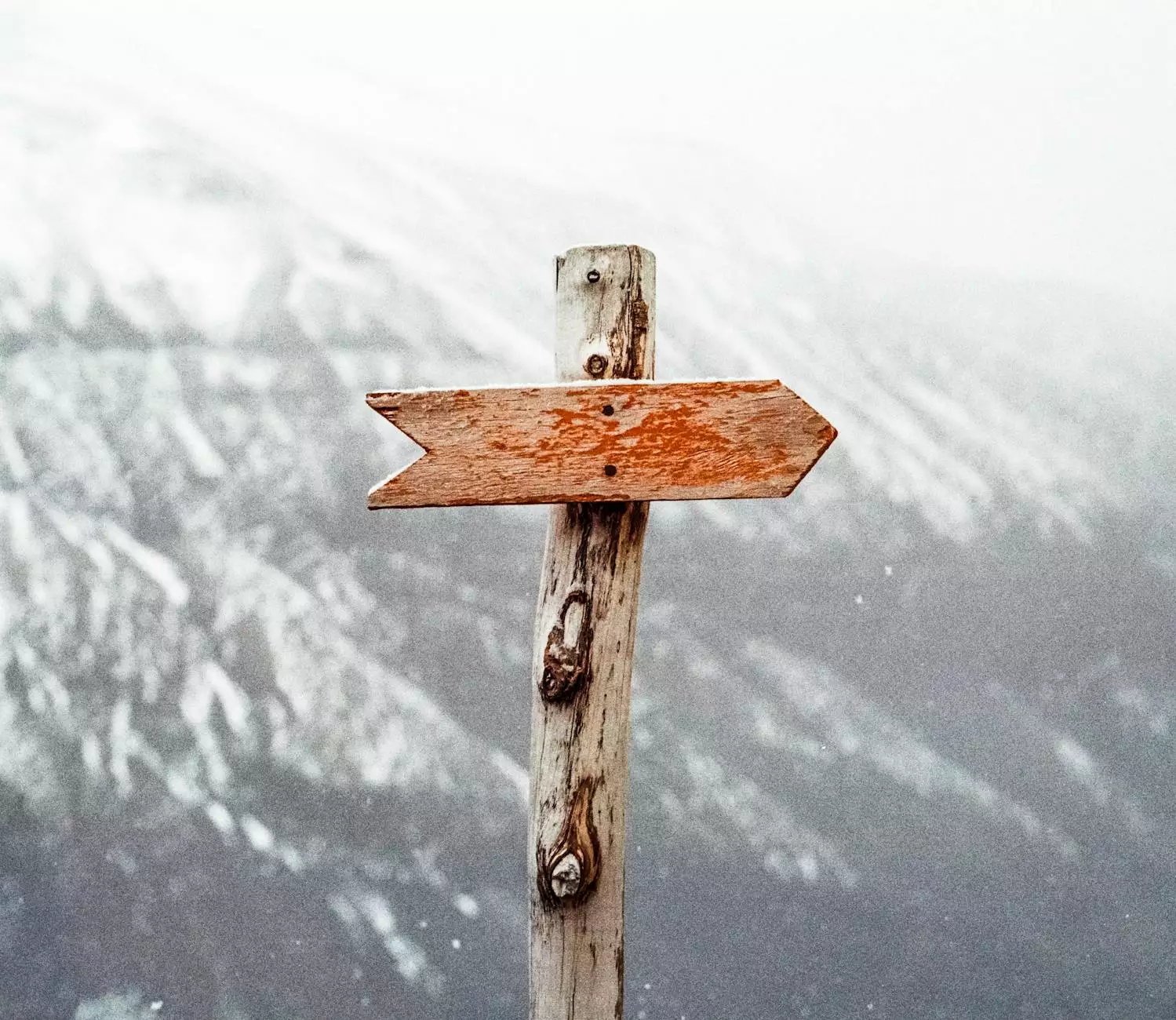
Introduction
Welcome to the ultimate guide on how to get a timestamp from a date! In this comprehensive article, we will explore various programming languages and their functionalities that allow you to extract timestamps from dates effortlessly. Whether you are a developer, web designer, or software enthusiast, this guide will equip you with the knowledge you need to manipulate and work with timestamps effectively.
Why Timestamps Matter
Timestamps play a crucial role in many applications and systems. They help in tracking events, measuring time differences, logging activities, and much more. Working with timestamps is essential in fields such as web design and software development, where precise timing is often required. Let's dive into the different programming languages and learn how to extract timestamps from dates using each one.
Python
Python is a versatile programming language that offers powerful libraries to handle dates and timestamps. One of the most commonly used libraries is the datetime module. By utilizing the datetime module, you can easily convert dates to timestamps and vice versa. The following Python code snippet demonstrates how to get a timestamp from a date:
import datetime date = datetime.date(2022, 9, 15) timestamp = datetime.datetime.timestamp(date) print("Timestamp:", timestamp)JavaScript
JavaScript is a popular programming language for web development that also provides functionalities to handle dates and timestamps. With JavaScript's native Date object, you can effortlessly obtain timestamps from dates. The following JavaScript code snippet showcases how to accomplish this:
const date = new Date('September 15, 2022'); const timestamp = date.getTime(); console.log("Timestamp:", timestamp);Java
Java, being a robust and widely used programming language, offers several built-in classes for managing dates and timestamps. The java.time package introduced in Java 8 simplifies working with dates and provides numerous methods for obtaining timestamps. The following Java code snippet demonstrates how to retrieve a timestamp from a date:
import java.time.LocalDate; import java.time.LocalDateTime; import java.time.ZoneOffset; LocalDate date = LocalDate.of(2022, 9, 15); LocalDateTime dateTime = date.atStartOfDay(); long timestamp = dateTime.toInstant(ZoneOffset.UTC).toEpochMilli(); System.out.println("Timestamp: " + timestamp);C++
C++ provides various libraries for handling dates and timestamps. The std::chrono library introduced in C++11 simplifies working with time-related operations and allows obtaining timestamps from dates. The following C++ code snippet showcases how to achieve this:
#include #include int main() { std::tm time{}; time.tm_year = 122; // Year is 1900-based time.tm_mon = 8; // Month is 0-based time.tm_mday = 15; std::time_t timestamp = std::mktime(&time); std::cout require 'time' date = Time.new(2022, 9, 15) timestamp = date.to_i puts "Timestamp: #{timestamp}"Conclusion
Congratulations! You have now learned how to extract timestamps from dates in various programming languages, including Python, JavaScript, Java, C++, and Ruby. Whether you are a web designer or a software developer, having this knowledge at your disposal will enable you to effortlessly work with timestamps and enhance your projects.
Remember that timestamps are essential in many applications and systems, and understanding how to handle them accurately is crucial for achieving precise time-based functionalities. With the knowledge gained from this guide, you are well-equipped to tackle any task involving timestamps in your web design or software development endeavors.
For further exploration and implementation, don't hesitate to refer to the official documentation of each programming language. Keep honing your skills, stay updated with the latest advancements, and achieve excellence in your projects!
get timestamp from date